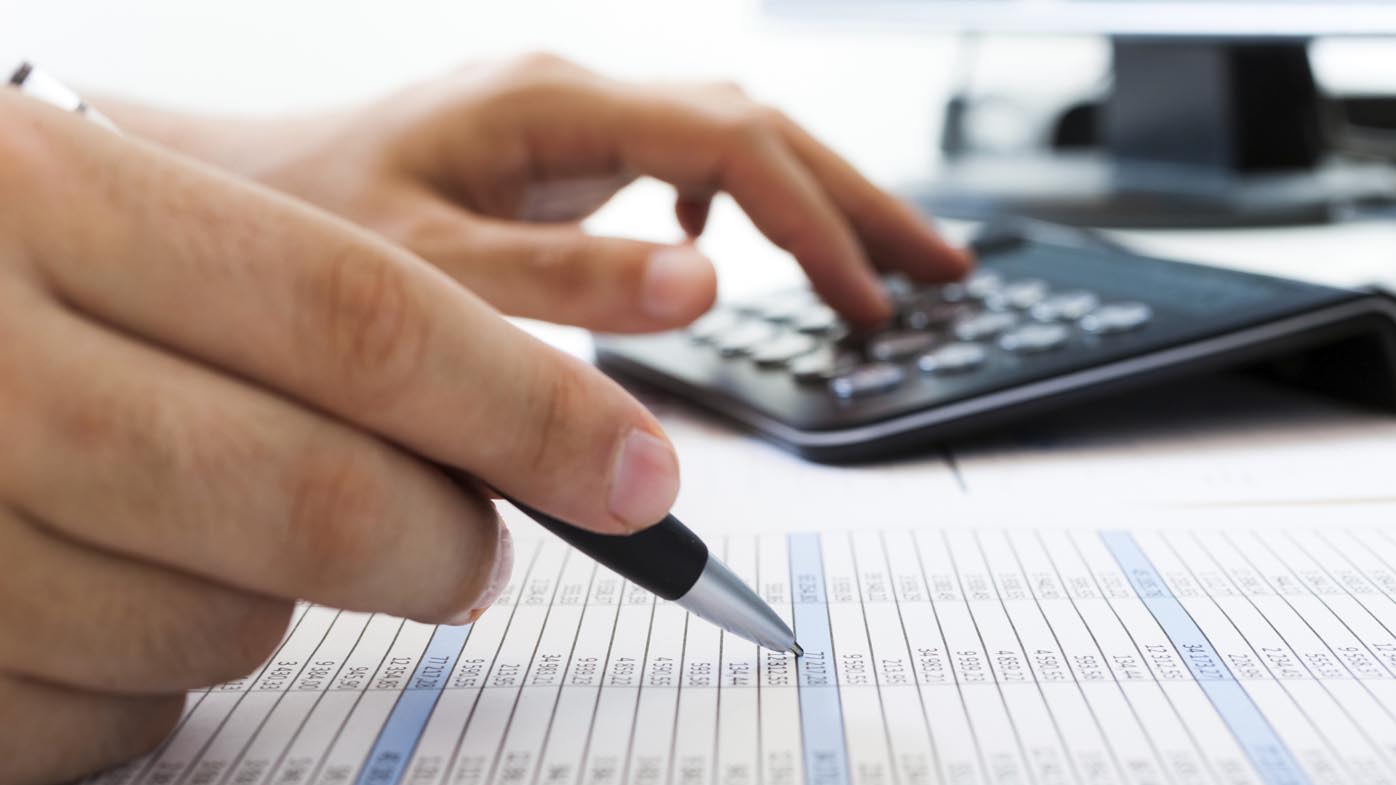
Tarvitsin tällaista ja etsiskelin sitä erilaisilta foorumeilta. Muutama koodinpätkä löytyi, mutta niidenkään alkuperäistä lähdettä ei kukaan muistanut. Ajattelin yhdistellä löytämäni tiedot ja tehdä yhden funktion, joka yhdistää kummatkin. Yleensähän viitenumero lasketaan joko asiakasnumeron tai laskun numeron perusteella, tms.
Tässä kirjalliset ohjeet suomalaisen viitenumeron laskemiseen
- Perusviitetieto kerrotaan vasemmalta oikealle luvuilla 1, 3, 7, 1, 3, 7 jne, kunnes viimeinen numero on kerrottu
- Saadut tulot lasketaan yhteen tarkistussummaksi
- Tarkistussummaa seuraavasta nollaan päättyvästä luvusta vähennetään tarkistussumma
- Erotus on viitenumeron tarkiste
Kirjallinen ohje ulkomaisen viitenumeron laskemiseen suomalaisesta
- Suomalaisen viitenumeron (huom! tarkisteineen, muuten se ei kelpaa suomalaisille pankeille) loppuun lisätään ”271500”
- Näin saatu luku jaetaan luvulla 97
- Jakolaskun jakojäännös (modulus) vähennetään luvusta 98, joka on tarkiste
- Kirjaimet RF ja tarkiste lisätään suomalaisen viitenumeron eteen
function viitenumero($in, $isrf, $print) {//$isrf = true/false onko kyseessä RF-viite, $print = true/false onko kyseessä tulostettava arvo, $in = strval($in);//muutetaan stringiksi, että päästään myöhemmin kertomaan numero kerrallaan $paino = array(7, 3, 1);//painoarvot, joita toistetaan kaikille luvun numeroille $summa = 0; for($i=strlen($in)-1, $j=0; $i>=0; $i--,$j++){ $summa += (int) $in[$i] * (int) $paino[$j%3];//lasketaan tarkistussumma viimeisestä ensimmäiseen siten, että jokainen luku kerrotaan järjestyksellä painoarvoin. } $tarkiste = (10-($summa%10)) % 10;//suomalaisen viitenumeron tarkiste $viite = $in.$tarkiste;
$modulus = 98 - intval($viite."271500") % 97;//jakojäännös $kvviite = "RF".$modulus.$viite;//kansainvälinen viitenumero if($isrf){ if($print){ return wordwrap($kvviite , 4 , ' ' , true );//jos tulostettava, lisätään lukemista helpottavat välilyönnit }else{ return $kvviite; } }else{ if($print){ return wordwrap($viite , 4 , ' ' , true ); }else{ return $viite; } } }
Huom! Tämä koodi on konsepti, jonka pitäisi toimia, mutta testatkaa ennen sen käyttämistä tuotantoympäristössä.
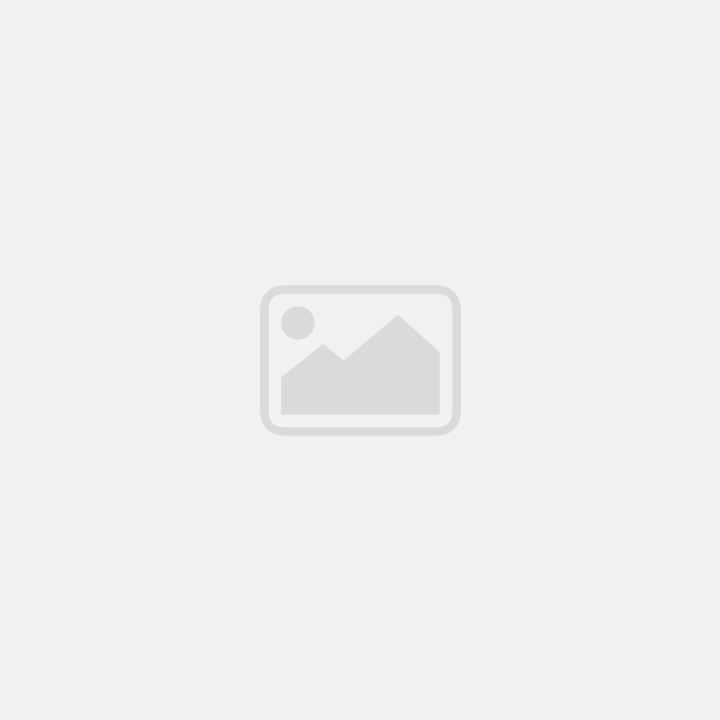
There are many plugins that implement a ”Nice Scrolling” functionality. This means that instead of the regular scrollbars, you will see a thinner one and the keyboard arrow keys can be configured to move the page one screen at a time. While this looks great on desktops and laptops, it is unecessary in mobile devices, that either have this functionality built in the browser or, as is the case of Windows Phone devices, simply cease scrolling altogether.
This is a problem for example in a very popular WordPress theme called Moesia. An easy way to fix it, is to edit hte functions.php and turn off nice scrolling for all mobile devices.
//Find the line that says 'moesia_scroller' in it, and add an AND NOT //clause using WordPress's inbuilt wp_is_mobile() function. //if ( get_theme_mod('moesia_scroller') != 1 ) { if ( (get_theme_mod('moesia_scroller') != 1) && !wp_is_mobile() ) {
For any other case, you will need to one of the following:
- disable the offending plugin (look for the word nice and scroll 😉 )
- contact the developer of your theme
- find a similar line as above and fix it yourself
- ask for help in such forums as Stackoverflow
I found this information at the WordPress support forums.
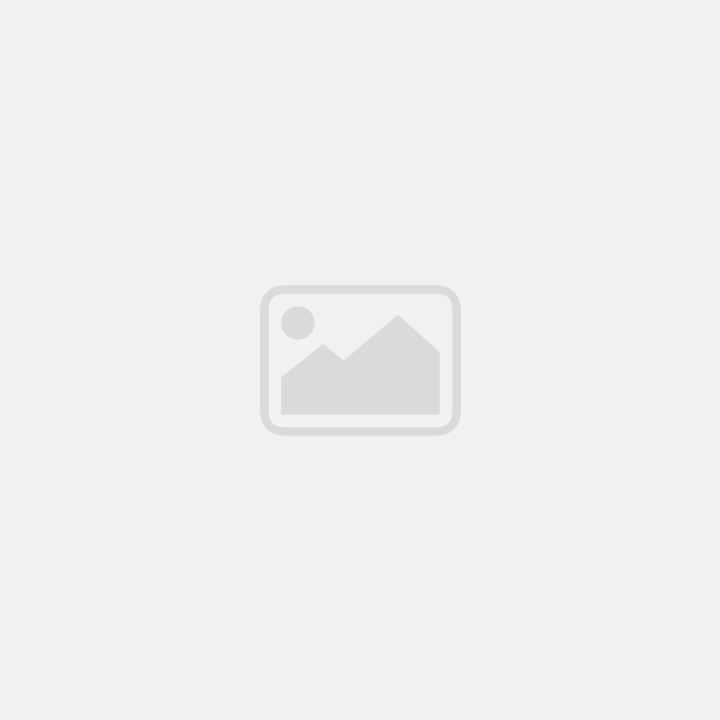
Sometimes, especially ’some’ mobile browsers do not respect the cache-headers you set in html meta tags or as htm headers with php. This is particularly annoiying, when your page or webapp has an error, like a typo or something, that does not get fixed on the client side unless they purge their browser caches manually (and why would they do that?) And in the case of a webapp, they cannot even do that.
So the only secure way is to always load the whole page from another file, adding a changing parameter to the URL so it really is different 😉 I have found this working nicely even in webapps installed on the user’s device. They pull the content nicely from a remote fileeverytime they open.
<!DOCTYPE HTML> <html> <head> <script language="Javascript"> var d = new Date(); var t = d.valueOf(); filename ="path_to_html_file?v="+t; var request = new XMLHttpRequest(); request.open("GET", filename, false); request.send(null); var CODE = request.responseText; document.write(CODE); document.close(); </script> </head> <!-- just in case ;-) --> <body style="background-color:black;"> </body> </html>
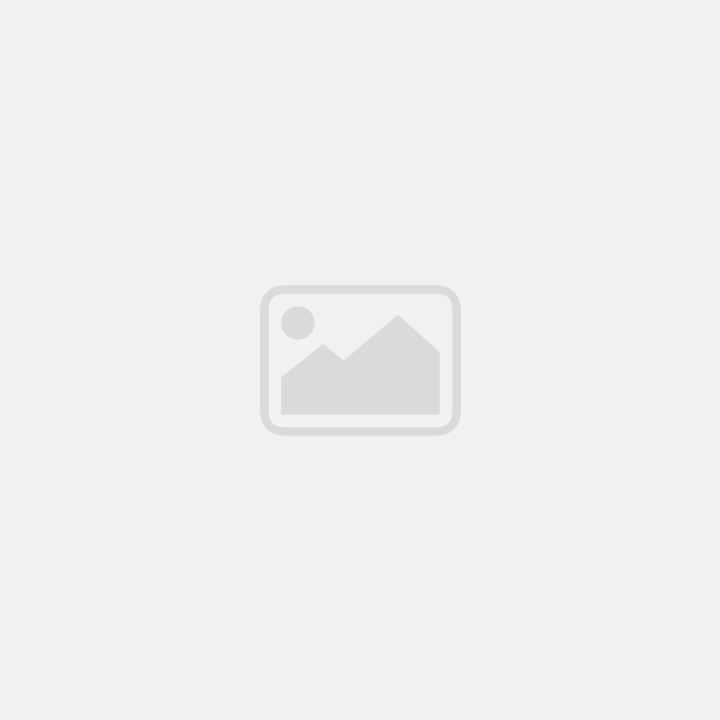
I started creating a sort of E-book in WordPress and could not find a good plugin for creating a clickable index in the beginning. So I baked my own. I created a page template that shows all articles in a certain category. So first you need to have a page and some articles in a category. This solution uses the <h1> and <h2> tags for the index. You can add more levels, if you wish.
This solution uses a PHP library called Simple HTML DOM for parsing the HTML and jQuery for JavaScript
PHP
This goes into the page template
<div id="tw_index"> <?php require_once( 'simple_html_dom.php' ); //use the ID of the category you wish to show $args = array( 'posts_per_page' : -1,'category' : 100,'orderby' : 'date', 'order' : 'ASC' ); $myposts = get_posts( $args ); $int = 1; //for numbering the main indexes foreach ( $myposts as $post ) : setup_postdata( $post ); //since the title is not in the content, it needs to be added and wrapped in a H1 //and a SPAN since we do not want the numbering included later on $html = str_get_html("<h1><span>$int. " . get_the_title() . "</h1>" . $post->post_content . "</span></h1>); foreach($html->find('h1,h2') as $h){ echo $h->outertext; } $int++; endforeach; ?> //And now the content <div id="tw_content"> <?php foreach ( $myposts as $post ) : setup_postdata( $post ); ?> <h1><?php the_title(); ?></h1> <p><?php the_content(); ?></p> <?php endforeach; wp_reset_postdata();?> </div>
JavaScript
The code below is added to the footer or header (the proper way of adding it)
//read only the text, not the index number, for <h1> jQuery("#tw_index h1 span").click(function(){tw_scrollTo('h1', jQuery(this).text());}); jQuery("#tw_index h2").click(function(){tw_scrollTo('h2', jQuery(this).text());}); function tw_scrollTo(el, elname){ var nAttr = "#tw_content " + el + ":contains('" + elname +"')"; jQuery('html, body').animate({//nicely animated ;-) scrollTop: jQuery(nAttr).offset().top -200 }, 1000); }
CSS
This goes to the styles.css so that the whole thing is more readable, also, since the clickable areas are not links, we need to change the cursor on hover and add a highlight.
div#tw_index h1{ padding:0px; font-size:20px; margin:20px 0 6px 0; color:#428bca; } div#tw_index h2{ padding:0px; font-size:16px; margin:0 0 6px 25px; color:#428bca; } div#tw_index h1:hover{ color:#e24871; cursor:pointer; } div#tw_index h2:hover{ color:#e24871; cursor:pointer; }